用例图描述图书管理
Building a Book Management System Using Lists
In the realm of software development, creating a book management system is a common yet pivotal task. Utilizing lists is a fundamental approach in programming, offering simplicity and efficiency. In this guide, we'll delve into the process of building a book management system using lists, from conceptualization to implementation.
1. Define Requirements:
Before diving into coding, it's crucial to outline the requirements of the book management system. Consider functionalities such as adding books, removing books, displaying the list of books, searching for books, and updating book details. This step ensures clarity and direction throughout the development process.
2. Design Data Structures:
Lists are versatile data structures for managing collections of items. In Python, you can utilize lists to store information about books. Each book can be represented as a dictionary containing attributes like title, author, publication year, and ISBN. The list itself can hold these dictionaries, forming a collection of books.
```python
books = [
{"title": "Python Programming", "author": "John Doe", "year": 2020, "isbn": "9780123456789"},
{"title": "Data Science Handbook", "author": "Jane Smith", "year": 2019, "isbn": "9780987654321"},
Add more books as needed
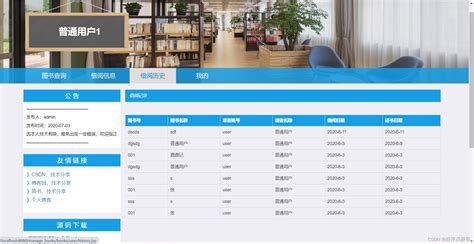
]
```
3. Implement Functions:
Next, implement functions to perform various operations on the book list. These functions will encapsulate the logic for adding, removing, searching, and updating books.
```python
def add_book(title, author, year, isbn):
books.append({"title": title, "author": author, "year": year, "isbn": isbn})
def remove_book(isbn):
for book in books:
if book["isbn"] == isbn:
books.remove(book)
break
def search_book(title):
for book in books:
if book["title"].lower() == title.lower():
return book
return None
Implement other functions as needed (e.g., display_books, update_book)
```
4. User Interface:
Create a user interface to interact with the book management system. This can be a commandline interface (CLI) or a graphical user interface (GUI) depending on your preferences and requirements. For simplicity, let's design a basic CLI.
```python
def main():
while True:
print("1. Add Book")
print("2. Remove Book")
print("3. Search Book")
print("4. Display All Books")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == "1":
title = input("Enter title: ")
author = input("Enter author: ")
year = int(input("Enter publication year: "))
isbn = input("Enter ISBN: ")
add_book(title, author, year, isbn)
elif choice == "2":
isbn = input("Enter ISBN of book to remove: ")
remove_book(isbn)
elif choice == "3":
title = input("Enter title of book to search: ")
book = search_book(title)
if book:
print("Book found:", book)
else:
print("Book not found.")
elif choice == "4":
display_books()
elif choice == "5":
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
```
5. Testing and Refinement:
Once the implementation is complete, thoroughly test the book management system to ensure it functions as expected. Test edge cases such as adding duplicate books, searching for nonexistent books, and updating book details. Refine the system based on feedback and identified issues.
6. Documentation and Maintenance:
Document the system including its functionalities, usage instructions, and any dependencies. Regularly maintain the system by addressing bugs, adding new features, and keeping dependencies uptodate.
Conclusion:
In conclusion, building a book management system using lists in Python provides a solid foundation for organizing and manipulating collections of books. By following the outlined steps, you can create a functional system tailored to your specific requirements, facilitating efficient book management.
版权声明
本文仅代表作者观点,不代表百度立场。
本文系作者授权百度百家发表,未经许可,不得转载。